Introduction
This article is meant to guide developers who are working with 123RF’s API towards successfully completing the integration of the API with any online system.
Table Of Contents
What you need to know
We will be using PHP to illustrate the examples.
The full API documentation can be found here: http://www.123rf.com/api
To proceed, you will need to apply for a Commercial API Key here: http://www.123rf.com/api/key/apply.php first
Then copy the API key that will be used in your app later on.
When you are ready to test your app before it goes live, you may ask your Account Manager to load some 123RF Download Credits into your API key for testing purposes.
Please understand that 123RF’s API is divided into 2 parts:
Non-commercial API The API calls that are listed here mainly deal with searching and filtering through the vast content library at 123RF and retrieval of content-based information such as contributors’ name, description of content and keywords associated with the content. These API methods are usually used to run search-based affiliate and referral programs to 123RF.com.
Commercial API 123RF’s Commercial API supports all the methods covered under the Non-commercial API. Generally, Developers use the Commercial API to develop website applications that support downloading of content and checking the amount of available credits that has been loaded into the Commercial API key.
This guide will concentrate on searching for content, as well as downloading content as an application that supports Print-On-Demand businesses.
Basic Requirements to Proceed with the API Implementation
Sign and Understand the Value Added Reseller Agreement
Please ensure that you have read and have managed to secure legal advise or have made an informed decision to agree with and abide by the terms and conditions as stipulated in the 123RF VAR Agreement.
123RF requires a signed copy of the agreement before we can approve your application to run a Commercial API Key.
A valid and approved Commercial API Key
Please apply for a Commercial API Key here : http://www.123rf.com/api/key/apply.php
We usually approve Commercial API key applications within 24 hours. If you do not received your approved Commercial API Key within the stipulated time, please get in touch with your Account Manager or contact us.
A valid Customer ID (CustID), SecretKey & AccessKey
Once your Commercial Key application has been approved, your Account Manager will supply you with your very own:
CustID – every Value Added Reseller will have their own unique CustID.
SecretKey – this is an important component as most commercial key API callbacks will require this SecretKey.
AccessKey – this is an important component as most commercial key API callbacks will require the AccessKey also.
Details of these 3 pieces of information can also be found on this page when it has been approved: http://www.123rf.com/api/key/list.php
Credits loaded into the API Key
For most of the API methods to work, you will need credits to be loaded into your API key. Without download credits, it would be impossible to test the download content method.
When your application is in the testing / sandbox phase, you may arrange for test credits to be loaded into your API key for testing purposes. Get in touch with your Account Manager to arrange for the test credits.
Code Examples
Some hotshot developers would be able to understand the API documentation ( http://www.123rf.com/api/documentation/ ) that we’ve prepared for you, however, if you need some examples to lead the way, we have prepared a few tutorials as well as some code examples to help you understand the implementation better.
The examples here are using a more procedural style of programming.
Part 2: Search & Display Search Results
Searching for images on 123RF can be made very easily. All we need is an APIkey.
About the Search API and the possibilities
123RF’s Search API (123rf.images.search) supports the following arguments:
As you can see from the table above, the API does a good job at allowing you to really dig deep into our content library. However, to keep things simple, we are going to use just a few of these possible arguments in our example.
Step 1: Prepare the Search Form
The example will concentrate on just a few of the possibilities highlighted above:
keyword is a must
order_by allows us to sort the results returned
perpage gives us some control over how many results will be returned when we search
media allows us to filter through some of the content types that we can search on 123RF.com
The HTML for the form is very simple:
<form method="get">
Keyword: <input type="text" name="keywords" size="35">
of <select name="orderby">
<option value="most_downloaded">Most Downloaded</option>
<option value="latest">Latest Images</option>
<option value="random">Random Order</option>
</select>
Show <select name="perpage">
<option value="20">20 items</option>
<option value="40">40 items</option>
<option value="60">60 items</option>
<option value="80">80 items</option>
<option value="100">100 items</option>
</select>
Media <select name="media_type">
<option value="all">All Types</option>
<option value="0">Photos</option>
<option value="1">Vectors</option>
<option value="4">Editorial</option>
</select>
<input type="submit" value="Search!">
</form>
Step 2: Processing the Parameters Passed to the Script and Constructing the API call
Now, we need to start constructing the URL that will be used in the API call. This is done by appending the results of the form into the URL string directly. No special URLencode function is required at this point.
<?php
// constants
$apikey = "fd022e333ae6ac4aa56ed1c3221cfb2c";
// extract arguments from $_GET
$keyword = $_GET['keywords'];
$perpage = $_GET['perpage'];
$orderby = $_GET['orderby'];
$media_type = $_GET['media_type'];
// for moving to another page
$page = $_GET['page'];
// construct the URL required for the API call
$theURL = "http://api.123rf.com/rest/?method=123rf.images.search&apikey=".$apikey.
"&keyword=".$keyword.
"&perpage=".$perpage.
"&orderby=".$orderby.
"&media_type=".$media_type.
"&page=".$page;
?>
Step 3 : Execute the API call and then display the results accordingly
Here we execute the API call and then we can display the thumbnails associated with it. To display the thumbnail, please refer to the documentation here: Thumbnail, but it’s actually very simple ; all URL to thumbnails take on this structure: http://images.assetsdelivery.com/thumbnails/[contributorid]/[folder]/[filename].jpg
<?php
// Execute the API call and store into a variable
$contents = file_get_contents($theURL);
// Declare this as a new SimpleXmlElement
$xml = new SimpleXmlElement($contents);
// Store the image results returned into an array
$imgs = $xml->images->image;
// Step through the array and output the results
foreach ($imgs as $item){
// prepare the thumbnail URL
$thumbnail = "http://images.assetsdelivery.com/thumbnails/$item[contributorid]/$item[folder]/$item[filename].jpg";
// output the result as the array is stepped through
echo <<< ___
<div style="float: left; margin: 10px 5px; height: 200px; width: 200px; text-align: center; vertical-align: bottom;">
<div>
<a href="#"><img src="$thumbnail" style="padding: 4px; border: #888888 solid 1px; background-color: #FFFFFF" alt="$item[description]"></a><br>
$item[id] © $item[contributorid]
</div>
</div>
___;
}
?>
Now, wasn’t that easy? You can enhance your script with code that makes the user experience better, but the above is the absolute minimum to get things working. Visit a demo of the 123RF Search API in action, or download the script above and experiment with it. Please note: you will have to insert your own API key into the script to get it to work!
Display Specific Content Details
This time around, we will be using looking at the description, keywords and larger size comping image for a specific 123RF Content ID.
About the GetInfo API
123RF’s GetInfo API (123rf.images.getInfo.V2) supports the following arguments.
This API call requires only 2 pieces of information to work, the content ID as well as the language. And if you are choosing English, you need not even supply this argument.
Step 1: Passing the Parameter and Value
In this example, we will just append a simple parameter and value to the script that we will create. Using the information, we will then get the API to furnish us with the XML that will enable us to display more information on the image.
The parameters passed will be imageID and language.
Say for example we are interested in this image’s details:
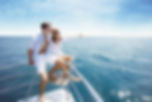
7362823 – couple relaxing at the tip of the yacht
The Image ID is 7362823. The language we are interested in is English.
Therefore, our parameter string will look like this: ?imageid=7362823&language=en
Step 2: Processing the Parameters Passed to the Script and Constructing the API call
The script has to start constructing the URL that will be used in the API call. This is done by passing the image ID and appending it into the API call URL string directly. No special URLencode function is required at this point.
<?php
// constants
$apikey = "fd022e333ae6ac4aa56ed1c3221cfb2c";
// extract arguments from $_GET
$imageid = $_GET['imageid'];
$language = $_GET['language'];
// construct the URL required for the API call
$theURL = "http://api.123rf.com/rest/?method=123rf.images.getInfo.V2&apikey=".$apikey.
"&imageid=".$imageid.
"&language=".$language;
?>
Step 3 : Execute the API call and then display the results accordingly
Here we execute the API call and then we can display the larger comp image. To display the comp image, please refer to the documentation here: Comping, but it’s actually very simple, all URL to thumbnails take on this structure: http://images.assetsdelivery.com/compings/[contributorid]/[folder]/[filename].jpg
<?php
// Execute the API call and store into a variable
$contents = file_get_contents($theURL);
// Declare this as a new SimpleXmlElement
$xml = new SimpleXmlElement($contents);
// retrieve the information specific to the image
$id = (string) $xml->image[id];
$dateuploaded = (string) $xml->image[dateuploaded];
$filename = (string) $xml->image[filename];
$folder = (string) $xml->image[folder];
$contributorid = (string) $xml->image->contributor[id];
$desc = $xml->image->description;
// construct the comping image URL
$compimg = "http://images.assetsdelivery.com/compings/$contributorid/$folder/$filename.jpg";
// Store all keywords into a variable and step through it to get all keywords into an array
$keywords = $xml->image->keywords->keyword;
foreach ($keywords AS $kw){
$keywordArr[$x++] = (string) $kw;
}
// Implode the keywords so that it looks like a normal string
$keywordsTxt = implode (", ", $keywordArr);
// Output the content's details for preview
echo <<< ___
<h1>$desc</h1>
<img src="$compimg">
<p>Keywords:<br />
$keywordsTxt
</p>
___;
?>
You can enhance your script with code that makes the user experience better, but the above is the absolute minimum to get things working. Visit a demo of the 123RF GetInfo API in action, or download the script above and experiment with it. Please note you will have to insert your own API key into the script to get it to work!
Checking Available Download Credits
Download credits are a necessary component of the entire Value Added Reseller program. When you sign up as a Value Added Reseller, you will have an assigned Customer ID. Any future reloads of purchased download credits will be associated with this Customer ID.
This segment deals with how we can use the 123RF API to check for available download credits in the assigned Customer ID.
About the getCreditCount API Method
123RF’s getCreditCount API method (123rf.customer.getCreditCount) requires the following arguments:
Please note that in order for this method to work you need to “sign” it with the “APIsign” method (API signature (apisign)). We’ll go through this tutorial so that you can understand the API sign better and how it works in the big scheme of things.
Step 1: Compiling the necessary information
In this example, we will be using 3 pieces of information:
Commercial API Key = b59e8b113748a3a0676045573eb667b2 Available from http://www.123rf.com/api/key/list.php – look under Commercial Key section
Secret Key = 8b5be6e0fad722 Available from http://www.123rf.com/api/key/list.php – look under Commercial Key section
Access Key = 44dacbef Available from http://www.123rf.com/api/key/list.php, click on the “Additional Info” link for the Commercial Key above.
Customer ID = b55310528064e5e Customer ID is given to you once you join the VAR program.
Now that we have gotten the necessary information, we can proceed to create the API sign which is another required argument to be sent to the API call.
Step 2: Generating the MD5 Hash for API Sign
In order to create the MD5 hash for API sign, we need to first determine the required arguments that have to be passed to the API call. This is the example that the documentation has given us: http://api.123rf.com/rest/? method=123rf.customer.getCreditCount&apikey=32c31c104fd62a1092c4af8f 922e5dd5&custid=46934e5f53ec7&accesskey=r7yu4hy65et4&apisign=y643y 7u865wghr6t4y6et68ujd564j88
It is then evident that we require :
apikey
custid
accesskey
apisign
Next, according to the APIsign documentation, we need to create the API signatures (apisigns) by using a combination of the secretkey and other arguments needed by API call in alphabetical order, argument name followed by the value.
The structure of the API signature looks like this: <secretKey>accesskey<accesskey>apikey<apikey>custid<custid>method<method> Plugging in the variables gives us this string:
8b5be6e0fad722accesskey44dacbefapikeyb59e8b113748a3a0676045573eb667b2custidb55310528064e5emethod123rf.customer.getCreditCount
Next, we need to pass this through a MD5 hash (using MySQL or PHP) to yield: dc94d733c8fe00f00e0944c22ebc4958
Step 3: Constructing the URL that will be passed to the API method
Now, we will construct the URL that the API call is expecting, and append the API sign at the back. We then have: http://api.123rf.com/rest/? method=123rf.customer.getCreditCount&apikey=32c31c104fd62a1092c4af8f 922e5dd5&custid=46934e5f53ec7&accesskey=r7yu4hy65et4&apisign=y643y 7u865wghr6t4y6et68ujd564j88
You may click on the link above to see that the XML returned looks something like this:
Therefore, if you have enough credits, you may now download the required content from within your application, which we will find out in the installment of the tutorial.
Downloading Content using the 123RF API
Now that we have checked and verified that we have sufficient download credits loaded into our Customer ID, we can start to download content from 123RF.com.
About the 123rf.images.download API Method
The 123rf.images.download API method requires the following arguments.
Please note that in order for this method to work, you need to “sign” it with the “APIsign” method (API signature (apisign)). We’ll take you through this tutorial so that you can understand the API sign better and how it works in the big scheme of things.
Step 1: Compiling the necessary information
In this example, we will be using 5 pieces of information…
Commercial API Key = b59e8b113748a3a0676045573eb667b2 Available from http://www.123rf.com/api/key/list.php – look under Commercial Key section
Secret Key = 8b5be6e0fad722 Available from http://www.123rf.com/api/key/list.php – look under Commercial Key section
Access Key = 44dacbef Available from http://www.123rf.com/api/key/list.php, click on the “Additional Info” link for the Commercial Key above.
Customer ID = b55310528064e5e Customer ID is given to you once you join the VAR program.
Resolution = blog The resolution of a file is very important. Do make sure that you choose the correct resolution that will meet the requirements of your intended use. For example, do not download an S size image for a 10 foot high wall decal.
Imageid = 7362823 This is just a reference image that I will be using throughout all my examples in the tutorial.
Now that we have gotten the necessary information, we can proceed to create the API sign which is another required argument to be sent to the API call …
Step 2: Generating the MD5 Hash for API Sign
In order to create the MD5 hash for API sign, we need to first determine the required arguments that have to be passed to the API call. This is the example that the documentation has given us: http://api.123rf.com/rest/? method=123rf.images.download.V2&accesskey=564e4173&apikey=567h78c 44fd62a1092c4af8f922e5dd5&custid=469484b1b4w2w&imageid=187887& resolution=high&apisign=0ee38590f4ba09999a8f01df8a5aa632
It is then evident that we require :
accesskey
apikey
custid
imageid
resolution
apisign
Next, according to the APIsign documentation we need to create the API signatures (apisigns) by using a combination of the secretkey and other arguments needed by API call in alphabetical order, argument name followed by the value.
The structure of the API signature looks like this <secretKey>accesskey<accesskey>apikey<apikey>custid<custid>imageid<imageid> method<method>resolution<resolution>
Please note that resolution comes after method because it is arranged ALPHABETICALLY!
Plugging in the variables gives us this string: 8b5be6e0fad722accesskey44dacbefapikeyb59e8b113748a3a0676045573eb 667b2custidb55310528064e5e imageid7362823method123rf.images.downloadresolutionblog
Next, we need to pass this through a MD5 hash (using MySQL or PHP) to yield 417130bb9a9e48c00f85915e695f8ca3
Step 3: Construct the URL that will be passed to the API method
We will now construct the URL that the API call is expecting and append the API sign at the back. This is what we get… http://api.123rf.com/rest/? method=123rf.images.download&accesskey=44dacbef&apikey=b59e8b1137 48a3a0676045573eb667b2&custid=b55310528064e5e&imageid=7362823&re solution=blog&apisign=417130bb9a9e48c00f85915e695f8ca3
When you use the link, you’ll see that the XML document will return something that looks like this:
The XML contains the link that you will have to click on to download the designated content from 123RF servers. Please note that when you call this API, the appropriate number of credits will be deducted from your account.